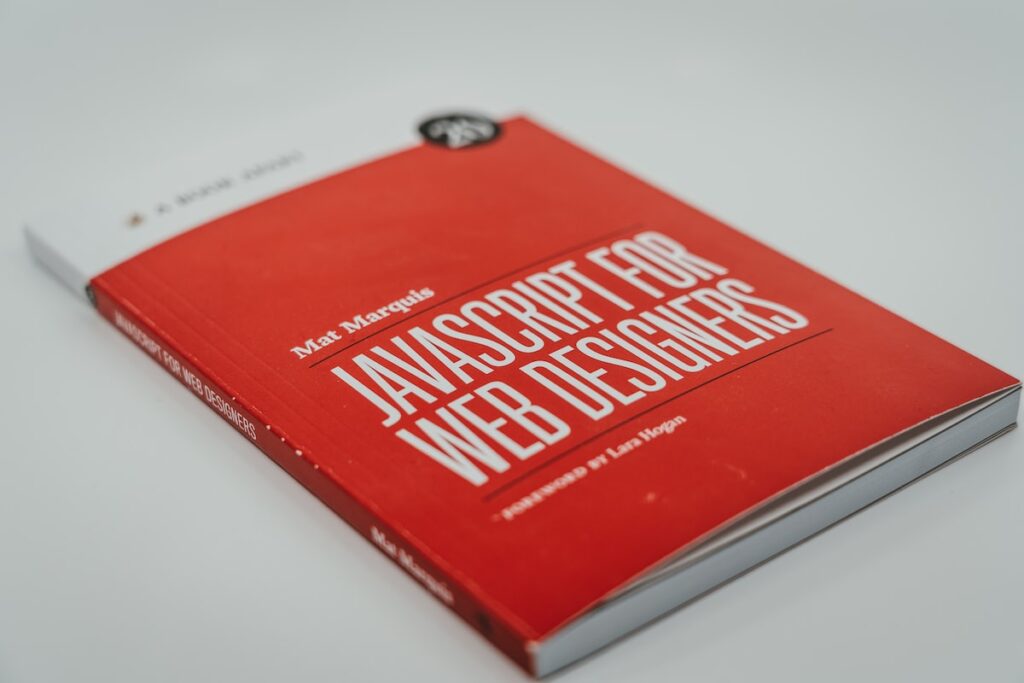
- What is JavaScript?
JavaScript is a programming language that is commonly used to create interactive effects within web browsers. It
is a client-side language, which means that it runs in the user’s web browser and not on the server. - What is the difference between JavaScript and Java?
Java and JavaScript are two completely different programming languages. Java is a high-level, object-oriented
language that is used to build standalone applications, while JavaScript is a scripting language that is used to
add interactivity to web pages. - What are the main features of JavaScript?
Some of the main features of JavaScript include:
- Support for object-oriented programming
- Support for imperative and functional programming styles
- Support for dynamic typing
- Built-in support for working with dates and times
- Support for regular expressions
- Support for working with the Document Object Model (DOM)
- How do you include a JavaScript file in a web page?
To include a JavaScript file in a web page, you can use the script tag with a src attribute that specifies the
path to the file. For example:
<script src=”path/to/javascript/file.js”></script>
- How do you add a JavaScript function to a web page?
To add a JavaScript function to a web page, you can define the function within the script tag and then call it
when you want to execute the code. For example:
<script>
function sayHello() {
alert(“Hello World!”);
}
</script>
To call the function, you can use an event handler or call it directly. For example:
<button onclick=”sayHello()”>Click me</button>
- What is an event in JavaScript?
An event in JavaScript is an action that occurs within the web page, such as a user clicking a button or moving
the mouse over an element. Events can be handled with event handlers, which are functions that are executed when
the event occurs. - What are the different types of events in JavaScript?
There are many different types of events in JavaScript, including:
- Mouse events, such as click and mouseover
- Keyboard events, such as keydown and keyup
- Form events, such as submit and change
- Window events, such as load and unload
- Drag and drop events
- What is an event handler in JavaScript?
An event handler is a function that is executed when a specific event occurs. Event handlers are typically used
to respond to user interactions and perform certain actions. - How do you create an event handler in JavaScript?
To create an event handler in JavaScript, you can use the following syntax:
element.event = function() {
// code to be executed
};
For example, to create a click event handler for a button element, you can use the following code:
document.getElementById(“myButton”).onclick = function() {
alert(“Button was clicked”);
};
- What is the DOM in JavaScript?
The DOM (Document Object Model) is a programming interface for HTML and XML documents. It represents the
structure of a document as a tree of objects, with each object representing an element in the document. The DOM
allows you to access and manipulate the elements of a document using JavaScript. - How do you access an element in the DOM in JavaScript?
To access an element in the DOM in JavaScript, you can use the document.getElementById() method. This method returns the element with the specified id attribute.Here's an example of how to access a paragraph element with the id "myParagraph":
let myParagraph = document.getElementById("myParagraph");
You can also use the querySelector() method to access elements by their tag name, class name, or other attributes. For example:
let myParagraph = document.querySelector("#myParagraph");
let myParagraphs = document.querySelectorAll(".myClass");
You can also use the document.getElementsByTagName() and document.getElementsByClassName() methods to access elements by their tag name or class name, respectively. These methods return a collection of elements, so you may need to access the specific element you want using an index. For example:
let myParagraphs = document.getElementsByTagName("p");
let firstParagraph = myParagraphs[0];let myElements = document.getElementsByClassName("myClass");
let firstElement = myElements[0];Finally, you can use the children property of an element to access its child elements. For example:
let myParent = document.getElementById("myParent");
let firstChild = myParent.children[0];